brainwagon (reborn)
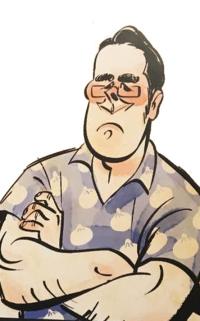
Welcome to the site of Mark VandeWettering.
Want to catch up with my blog? Click here and get all the latest. Or use RSS to stay up to date.
This site is generated using a modified version of Sunaina Pai's makesite.py Python script. My modified version generates this blog from Markdown source using under 1000 lines of very comfortable Python. Her script is just 250 lines, and could be a good start for someone wanting to ditch large systems for blogging.
In any case, if you are reading this and have any comments or questions, feel free to send me an email and I'll try to respond. My goal is to eventually write up a more complete HOWTO on how I created this.