Okay, so yesterday I did some sprite animation and learned a bit about how color maps work on the Gameduino. I didn’t have a lot of time to tinker with the Gameduino tonight, but I did want to test my understanding of sprites, so I made a simple little program to display my brainwagon logo (blue Mark and his wagon o’ brains) on the left using the Arduino.
There are a few things that you have to understand.
First, there is memory for 64 sprite images, each one being 16×16 pixels. But that’s if you use 8 bit colormaps. If you choose to just use 4 bit maps, you can get twice as many. I rescaled my logo to be 96×128 pixels (a convenient multiple of 16) and used the netpbm utilities to convert it down to just 16 colors. Normally, that would be 6*8 = 48 sprite images, but since we are just using 16 colors, we end up with using 24 sprites. I wrote a shell script and a small C program to use the netpbm to dump the raw bits, and then used John’s python “compress” function to compress the array into an array that can be stored in flash memory. The net result is the following display, followed by the code used to display it.
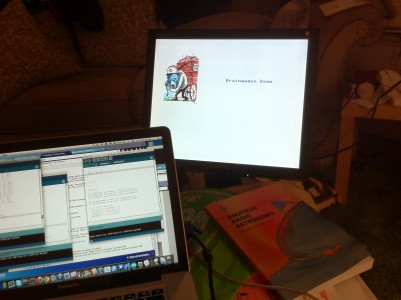
[sourcecode lang=”C++”]
#include
#include
#include
static PROGMEM prog_uchar data[] = {
0xc1, 0x21, 0x20, 0x8a, 0x10, 0x61, 0x22, 0xc0, 0xcc, 0x04, 0x44, 0x20,
0x84, 0x7d, 0x18, 0x86,
0x63, 0x04, 0x9a, 0x0a, 0xe4, 0x08, 0x35, 0x93, 0x0e, 0xa2, 0x4c, 0x05,
0x90, 0x61, 0xc1, 0x86,
0x73, 0x18, 0x87, 0x08, 0xc1, 0xa1, 0x11, 0x11, 0xa2, 0xc2, 0x33, 0x80,
0x71, 0xe0, 0xc0, 0x85,
0x39, 0x00, 0x70, 0x05, 0xa0, 0x28, 0x51, 0x82, 0x03, 0x10, 0x0f, 0x8e,
0x70, 0x8d, 0xc0, 0xf0,
0xc6, 0x13, 0x4f, 0x01, 0x1d, 0x03, 0x82, 0x03, 0x22, 0xbc, 0x13, 0x38,
0xb8, 0x52, 0x23, 0xbd,
0x33, 0x34, 0xbc, 0xe3, 0x3f, 0x53, 0xd4, 0xa4, 0x77, 0x46, 0x25, 0x51,
0xfc, 0x45, 0xc8, 0x40,
0x62, 0xc6, 0x11, 0xff, 0xe1, 0xd0, 0x1e, 0xf1, 0xdf, 0x04, 0xb1, 0x80,
0x59, 0x54, 0xfa, 0x07,
0x3e, 0x66, 0x31, 0x01, 0xf0, 0xf9, 0x21, 0x24, 0xe6, 0x0b, 0x23, 0x3c,
0x7c, 0x22, 0xf2, 0x2b,
0xe2, 0x5f, 0xc4, 0x07, 0x3c, 0x87, 0xe0, 0xa0, 0x10, 0x21, 0xea, 0x2b,
0xdf, 0x42, 0x85, 0x08,
0x1c, 0x11, 0x64, 0xf8, 0x2d, 0x44, 0xcd, 0xf7, 0x7f, 0x04, 0xc4, 0x75,
0x36, 0x7e, 0x38, 0x7d,
0x50, 0x0c, 0x5c, 0x8e, 0x8a, 0xfb, 0xfe, 0x18, 0x55, 0x01, 0xa8, 0x39,
0xdc, 0x65, 0xe9, 0xd2,
0x85, 0x2a, 0x22, 0x1c, 0x13, 0x0e, 0x17, 0x55, 0xb0, 0x60, 0xf1, 0x42,
0xa5, 0x0b, 0xa6, 0x6e,
0xc9, 0xf1, 0xaa, 0x0b, 0x16, 0xa8, 0x00, 0xe4, 0xcd, 0x34, 0x6d, 0x64,
0xa1, 0xa2, 0xc5, 0x0b,
0x14, 0x10, 0x50, 0x8c, 0x79, 0x71, 0x29, 0x07, 0xc5, 0x01, 0xa0, 0x0b,
0x0a, 0x17, 0x26, 0x4c,
0x78, 0x59, 0xb6, 0xcc, 0x18, 0x30, 0xe0, 0xb0, 0x54, 0xac, 0x19, 0x0a,
0x90, 0xd8, 0x60, 0x65,
0x8b, 0xb1, 0xe5, 0x70, 0x00, 0x15, 0x1e, 0xaa, 0x14, 0x53, 0xb6, 0x62,
0x8b, 0x15, 0x67, 0xce,
0x80, 0x79, 0xb1, 0xaa, 0x0c, 0x58, 0x90, 0x21, 0x43, 0x61, 0x42, 0x25,
0x37, 0xe2, 0xc2, 0x96,
0xe5, 0x42, 0x04, 0xc5, 0xfa, 0xdf, 0x83, 0xe9, 0x0a, 0x54, 0x84, 0xc7,
0x4d, 0x84, 0x4e, 0x74,
0x89, 0x12, 0x11, 0x1f, 0x1f, 0x91, 0xd0, 0xa6, 0xa2, 0x2b, 0x3e, 0xca,
0x63, 0x03, 0xde, 0x2b,
0x00, 0x04, 0x7f, 0xa2, 0xe0, 0x97, 0x89, 0x60, 0x51, 0x46, 0x4c, 0x86,
0x83, 0xe0, 0x78, 0x7c,
0x4b, 0x51, 0xa6, 0x0c, 0x9b, 0x32, 0x6a, 0xd4, 0x74, 0x42, 0x2a, 0xfe,
0xc5, 0x7d, 0x4c, 0x0d,
0x53, 0xa6, 0xc3, 0x49, 0xd4, 0x67, 0xbc, 0xa2, 0xa8, 0x08, 0x0a, 0x98,
0xf0, 0x08, 0xc8, 0xa0,
0x8a, 0xcc, 0x28, 0x82, 0x00, 0x4b, 0x57, 0x24, 0x27, 0xb7, 0x00, 0x81,
0x0a, 0x81, 0x02, 0xd0,
0x0d, 0x51, 0xa3, 0x42, 0xd5, 0x18, 0x31, 0xaa, 0xc6, 0x14, 0x0e, 0x42,
0x45, 0x2b, 0xe0, 0xc6,
0x94, 0x35, 0x07, 0x08, 0xda, 0x95, 0x62, 0xc7, 0x80, 0x1d, 0x23, 0x6e,
0x8c, 0xa2, 0x85, 0x0b,
0xa7, 0xce, 0x14, 0x00, 0x00, 0x60, 0xd0, 0x94, 0xa9, 0xc5, 0x86, 0x17,
0x2f, 0x56, 0xa5, 0x02,
0x85, 0x09, 0xa4, 0x0e, 0x95, 0x1b, 0x30, 0x60, 0xda, 0xa1, 0x2b, 0x8f,
0x08, 0xe5, 0x4a, 0x95,
0xc6, 0x83, 0x30, 0xae, 0xcc, 0xa8, 0x59, 0xe5, 0x0e, 0x1c, 0x3a, 0x42,
0xa4, 0xc0, 0x94, 0x29,
0x15, 0x26, 0x5c, 0xac, 0x30, 0x60, 0x00, 0x34, 0x40, 0xe7, 0xca, 0x8d,
0x2a, 0x5c, 0x6d, 0xd0,
0xb4, 0x2b, 0x0f, 0x07, 0xe1, 0x02, 0xf0, 0xe0, 0x39, 0xe8, 0x40, 0x0f,
0xff, 0xf0, 0xc3, 0x11,
0xff, 0xe1, 0x9f, 0x31, 0x86, 0xe3, 0xbf, 0xe0, 0xf3, 0x17, 0x0b, 0x96,
0xca, 0x2f, 0xff, 0xfa,
0x6f, 0xff, 0xf6, 0x6b, 0xf0, 0xf0, 0xcb, 0xfb, 0xf0, 0x5f, 0xf4, 0xf8,
0xdf, 0xf2, 0xfe, 0xc0,
0x13, 0x7e, 0x82, 0x4f, 0x52, 0xf2, 0x79, 0x84, 0x9f, 0xe0, 0x00, 0x54,
0x0c, 0x38, 0x3c, 0x43,
0x25, 0x70, 0xc2, 0x43, 0x8c, 0x99, 0xb0, 0x57, 0x1c, 0x9f, 0x01, 0xc0,
0xc5, 0xb2, 0x2d, 0xc6,
0x8c, 0x39, 0x83, 0xe2, 0x45, 0x8b, 0x17, 0x28, 0x50, 0xa0, 0x01, 0x72,
0x66, 0x4c, 0x39, 0x2c,
0x02, 0x93, 0x17, 0x2c, 0xd6, 0xa0, 0x01, 0x01, 0x00, 0xca, 0xdd, 0xb2,
0x63, 0xce, 0x80, 0x41,
0x01, 0xe6, 0xec, 0xca, 0x96, 0x6b, 0xae, 0xdc, 0xb9, 0x71, 0x65, 0xca,
0x37, 0x2e, 0x32, 0x91,
0x73, 0x78, 0xb0, 0xe6, 0x0a, 0x0c, 0x00, 0x07, 0x6b, 0x60, 0x81, 0xc7,
0x63, 0x26, 0x70, 0xb6,
0xc5, 0x10, 0x20, 0x70, 0x0e, 0x1c, 0x38, 0x5a, 0x65, 0xc6, 0x3c, 0x1e,
0x39, 0x03, 0x01, 0x19,
0x17, 0xdc, 0xd9, 0x72, 0x07, 0x96, 0x10, 0xc0, 0xd9, 0xc6, 0x03, 0x63,
0xd6, 0x0c, 0x6d, 0x5b,
0x64, 0xc7, 0xd7, 0x3a, 0xf7, 0x44, 0x00, 0xc0, 0xcd, 0x5a, 0x5a, 0xf0,
0x06, 0x96, 0x12, 0xd6,
0xf8, 0xf2, 0xe5, 0xca, 0x1c, 0x22, 0x75, 0x07, 0xce, 0x1c, 0xf4, 0x07,
0x57, 0x0e, 0x0c, 0x28,
0xb8, 0x75, 0xe0, 0xdc, 0x99, 0x43, 0xab, 0xd6, 0x2c, 0x04, 0x00, 0x65,
0x5a, 0xee, 0x1c, 0x44,
0x83, 0x75, 0x06, 0x0c, 0x2c, 0x94, 0x07, 0x07, 0xe6, 0xd6, 0xac, 0xcd,
0x81, 0x3d, 0x0b, 0xd6,
0x2d, 0x70, 0x67, 0xce, 0x90, 0x69, 0x1f, 0xd0, 0xe3, 0x67, 0xcf, 0x9e,
0x25, 0xdb, 0xf6, 0x2c,
0x32, 0xe5, 0x47, 0x0f, 0x9e, 0x3e, 0xb8, 0x70, 0xe9, 0xd2, 0xb5, 0xcb,
0xd6, 0x2e, 0x3b, 0xab,
0x41, 0x10, 0x14, 0xa1, 0x40, 0x97, 0x6d, 0x04, 0xc2, 0xad, 0x8f, 0x22,
0x08, 0x4f, 0xb8, 0xce,
0xd8, 0xd2, 0xa5, 0x46, 0xd7, 0x99, 0x5d, 0x66, 0xcc, 0xec, 0x86, 0x07,
0x5b, 0x66, 0x6c, 0x9d,
0x59, 0x73, 0xeb, 0x36, 0x1d, 0x92, 0x83, 0x1b, 0x37, 0x66, 0xf1, 0x11,
0x9a, 0x74, 0x03, 0x01,
0x5a, 0x7c, 0x70, 0xe3, 0x1b, 0x0e, 0x6c, 0x81, 0x71, 0x67, 0xe6, 0x8c,
0x1b, 0x3b, 0x86, 0xc0,
0x80, 0x03, 0xd7, 0x0e, 0x4d, 0x83, 0x70, 0xe1, 0x02, 0xd2, 0x02, 0x38,
0x75, 0x6e, 0xfc, 0xf8,
0x32, 0x4b, 0x06, 0xe6, 0x91, 0x20, 0x4c, 0x98, 0x5a, 0x66, 0xc0, 0x46,
0x02, 0x1a, 0xc3, 0x19,
0xf8, 0x03, 0x0e, 0x00, 0xe2, 0x51, 0xc0, 0x42, 0x7c, 0x01, 0xd8, 0x0a,
0x58, 0x04, 0x6e, 0xc0,
0x40, 0x2b, 0x30, 0x60, 0x4c, 0x2c, 0x70, 0x6e, 0x6c, 0x01, 0x04, 0x00,
0x3b, 0x8e, 0x1c, 0xa1,
0x69, 0x03, 0xaa, 0x56, 0xac, 0x30, 0x01, 0x95, 0xf1, 0x14, 0xdf, 0x00,
0x8e, 0x1b, 0x50, 0x80,
0x0c, 0xb5, 0x46, 0x80, 0x68, 0xd1, 0xce, 0x9c, 0x39, 0x75, 0x17, 0x00,
0x54, 0xe9, 0x41, 0x63,
0x0a, 0x0d, 0x1e, 0x5f, 0x75, 0xe2, 0xc4, 0xd9, 0xb3, 0x1b, 0xc0, 0xfc,
0x56, 0x70, 0x3b, 0xc0,
0x8a, 0x15, 0xbb, 0x18, 0x6c, 0xad, 0x71, 0x0f, 0x04, 0xbe, 0xd6, 0x98,
0x72, 0x03, 0x08, 0xb6,
0x03, 0xcc, 0x5a, 0xb3, 0x9e, 0x00, 0x6c, 0x99, 0x5b, 0x67, 0xc6, 0x94,
0x29, 0x30, 0xa0, 0xc0,
0x80, 0x99, 0x35, 0x7b, 0x96, 0x01, 0x11, 0xde, 0xcc, 0xb8, 0x2e, 0x81,
0x51, 0xa3, 0x60, 0xb9,
0x6b, 0xd3, 0xa6, 0x3d, 0x00, 0xb4, 0x69, 0xd5, 0xaa, 0x9d, 0x6a, 0x78,
0x08, 0x14, 0xc4, 0x56,
0x9b, 0xde, 0x08, 0xe0, 0x86, 0x8c, 0x5b, 0x78, 0xa0, 0x0e, 0x0f, 0xd0,
0x0a, 0x0d, 0x82, 0x05,
0xa6, 0x56, 0x99, 0x72, 0xe4, 0xc8, 0x81, 0x2b, 0x05, 0xaa, 0x0d, 0x22,
0x70, 0x65, 0x02, 0x45,
0x67, 0x06, 0x30, 0xe5, 0x01, 0xa8, 0x4c, 0x29, 0x52, 0xa4, 0xf0, 0xb4,
0x2a, 0x0d, 0x0f, 0x6d,
0x1a, 0x73, 0x42, 0x2b, 0xc7, 0x20, 0xc8, 0x06, 0xe4, 0x50, 0x91, 0xc5,
0xa7, 0x19, 0x31, 0x62,
0x04, 0x07, 0x90, 0xe2, 0x00, 0x08, 0x2e, 0x35, 0xe1, 0xa0, 0x26, 0x0c,
0x01, 0x23, 0xd6, 0x1c,
0x00, 0x88, 0x15, 0x8b, 0x11, 0xa1, 0x22, 0x1c, 0xf4, 0x68, 0xa6, 0xec,
0x98, 0x32, 0x65, 0xc8,
0x01, 0x08, 0x03, 0x86, 0x0c, 0x15, 0x70, 0xdc, 0xd0, 0x81, 0x1c, 0x1b,
0x9c, 0xe3, 0x51, 0x07,
0x0f, 0x10, 0x3c, 0x68, 0xe9, 0x86, 0xa3, 0x47, 0x47, 0x2a, 0x86, 0x53,
0x00, 0xe8, 0xe4, 0x08,
0x0e, 0x2a, 0x0f, 0x30, 0x88, 0xe3, 0x20, 0x1e, 0xc3, 0x41, 0x97, 0x46,
0x7d, 0x1a, 0x75, 0x83,
0xe6, 0xa3, 0x87, 0x33, 0xe0, 0x00, 0xe4, 0xc1, 0x83, 0x17, 0x47, 0x7e,
0xbc, 0x79, 0x73, 0xd2,
0xa0, 0x46, 0x45, 0x3b, 0x89, 0x10, 0xa1, 0x0a, 0x34, 0x38, 0x8e, 0x9c,
0x38, 0x70, 0xd0, 0xd5,
0x08, 0x22, 0xc2, 0x51, 0x54, 0x00, 0x00, 0x05, 0x0a, 0x80, 0x57, 0x00,
0xe8, 0x50, 0x11, 0x1e,
0x8a, 0x55, 0x04, 0x80, 0x2a, 0xd5, 0xe0, 0x14, 0xa8, 0x02, 0x05, 0x8a,
0x97, 0x2e, 0x3d, 0x7a,
0x50, 0x24, 0x44, 0x3c, 0x0a, 0x55, 0x0d, 0x2a, 0x3c, 0x0b, 0x02, 0x06,
0x0f, 0x18, 0x3a, 0x22,
0x50, 0xa0, 0x50, 0x55, 0xf1, 0xa0, 0x4a, 0x21, 0x00, 0xe0, 0x7c, 0x86,
0x82, 0x86, 0x2a, 0x51,
0x82, 0xc4, 0x09, 0x0a, 0x8f, 0x70, 0x00, 0xfa, 0x16, 0x2d, 0xd0, 0x93,
0x69, 0x21, 0x5a, 0x54,
0x21, 0x28, 0x54, 0xa6, 0x4c, 0x39, 0x70, 0x16, 0x2c, 0x40, 0x81, 0x80,
0x25, 0xd0, 0xa8, 0x29,
0x53, 0xe1, 0xa5, 0x5c, 0xb9, 0x6a, 0x30, 0x3c, 0x54, 0x80, 0xa0, 0x25,
0xd0, 0x60, 0x78, 0x0c,
0x44, 0x78, 0x2a, 0x03, 0x66, 0x91, 0xad, 0x5b, 0xba, 0xce, 0x9c, 0x41,
0xe3, 0x06, 0x0d, 0x9a,
0x5e, 0xb8, 0x74, 0xdd, 0x3a, 0xa3, 0x46, 0xdb, 0xa9, 0xb5, 0x70, 0x70,
0x16, 0x52, 0x01, 0x60,
0x97, 0xa9, 0x55, 0x6b, 0xae, 0x9d, 0x86, 0x04, 0x34, 0x6d, 0xdc, 0xc0,
0xce, 0x03, 0x38, 0x7b,
0xf6, 0xec, 0xda, 0xb6, 0xe7, 0xc0, 0x9d, 0x56, 0xae, 0x21, 0x00, 0x90,
0x23, 0x30, 0x60, 0xdc,
0xac, 0xbb, 0x75, 0xea, 0xce, 0x1d, 0x44, 0xd0, 0xbc, 0x6d, 0x67, 0x03,
0x05, 0x07, 0x14, 0xdc,
0xba, 0x85, 0x6e, 0x95, 0x9d, 0x3d, 0x76, 0x14, 0x1d, 0xba, 0x72, 0x6e,
0xd7, 0x82, 0x05, 0xeb,
0xb6, 0xed, 0x5a, 0xe5, 0xc7, 0x8e, 0x5f, 0x26, 0x58, 0xb7, 0x67, 0xd1,
0x22, 0xd3, 0x9c, 0xb0,
0xc7, 0x36, 0x08, 0xe2, 0xa2, 0x00, 0x0a, 0x82, 0x35, 0x53, 0x6b, 0x6c,
0x83, 0xc1, 0x2e, 0xbb,
0xd4, 0x64, 0x1b, 0x00, 0x7c, 0x6d, 0x5b, 0x67, 0x67, 0x37, 0x38, 0xe3,
0x80, 0x9d, 0xd5, 0x70,
0xb4, 0x6b, 0x8d, 0xb5, 0x75, 0x86, 0x56, 0x99, 0x31, 0x4d, 0xe5, 0xe8,
0x62, 0xc6, 0xda, 0x76,
0x67, 0x54, 0x47, 0x1a, 0x24, 0x66, 0x18, 0xf0, 0xe5, 0xc6, 0x2d, 0x1c,
0x18, 0xa4, 0x66, 0x41,
0xa4, 0x51, 0x71, 0xc5, 0x87, 0x05, 0xab, 0xa9, 0xb5, 0x1a, 0x4c, 0x99,
0x65, 0x07, 0x53, 0xb6,
0xb4, 0x59, 0x33, 0x68, 0x88, 0x90, 0xe2, 0xcd, 0x2a, 0x00, 0x42, 0x01,
0x2a, 0x74, 0xd7, 0x14,
0x68, 0xbb, 0xa3, 0xe8, 0x94, 0x36, 0x05, 0x0a, 0xb4, 0x02, 0x22, 0x12,
0x6c, 0x29, 0x47, 0x46,
0xdd, 0xc1, 0x24, 0xd8, 0x02, 0x05, 0x00, 0x00, 0x07, 0x0b, 0x0c, 0x38,
0x84, 0x87, 0x46, 0xde,
0xba, 0xa1, 0x43, 0xd0, 0x06, 0x01, 0xa2, 0x36, 0x6d, 0xc0, 0x21, 0xea,
0x55, 0x2b, 0x54, 0x34,
0x72, 0x00, 0x0a, 0x80, 0x73, 0xe0, 0xc6, 0x41, 0x63, 0x78, 0xe9, 0x56,
0x1d, 0x1e, 0xc8, 0x94,
0x01, 0xfb, 0x85, 0xdf, 0xe0, 0x07, 0x4e, 0x1f, 0x30, 0xa8, 0x5a, 0x15,
0x0a, 0x15, 0xa4, 0x2f,
0x29, 0xe0, 0xea, 0x05, 0xab, 0x17, 0xb8, 0x56, 0x8d, 0xe1, 0xa9, 0x48,
0x9c, 0x00, 0xbd, 0xd0,
0x99, 0x43, 0x8b, 0x00, 0xe8, 0xda, 0xa1, 0x42, 0x53, 0x08, 0x95, 0xab,
0x02, 0x71, 0xa2, 0xf4,
0x72, 0x03, 0xc6, 0x0d, 0x2c, 0x30, 0x6d, 0xc8, 0xb5, 0x86, 0x27, 0xd2,
0xf0, 0x02, 0x06, 0x0c,
0x38, 0x58, 0xe0, 0x6a, 0x81, 0x03, 0xd5, 0xa6, 0x94, 0x2a, 0x52, 0xe5,
0xea, 0x44, 0x35, 0x07,
0x77, 0x6e, 0xc7, 0xa8, 0x5d, 0xab, 0x36, 0xa0, 0x4e, 0x01, 0xaa, 0x4e,
0x4f, 0xbd, 0xda, 0xa8,
0x05, 0x82, 0x5e, 0x68, 0x40, 0x99, 0x01, 0x75, 0x18, 0x9e, 0x02, 0xb4,
0x21, 0x03, 0xce, 0x3d,
0x3f, 0xc0, 0x72, 0xe3, 0xaa, 0x8d, 0xab, 0x33, 0x85, 0xe1, 0x55, 0x0e,
0x71, 0x11, 0x10, 0xda,
0xc1, 0x6a, 0xe3, 0xe7, 0x0c, 0xa0, 0xc2, 0xf6, 0x54, 0x0b, 0x2c, 0x13,
0xc3, 0x03, 0x18, 0x43,
0xa0, 0xd4, 0x01, 0x2a, 0x55, 0x2a, 0x28, 0x3e, 0x16, 0xa0, 0x21, 0x02,
0x80, 0x1b, 0x53, 0xa0,
0x14, 0x80, 0x2a, 0x15, 0xaa, 0x5a, 0xab, 0xd2, 0xa0, 0x08, 0x80, 0x4d,
0xe0, 0xa7, 0x15, 0x28,
0x04, 0x84, 0x6a, 0xd5, 0xa9, 0xae, 0x4d, 0x6d, 0xd0, 0x35, 0x04, 0x80,
0x46, 0x68, 0xc0, 0x20,
0x68, 0x0c, 0x4f, 0x6a, 0xd4, 0xe1, 0x42, 0xdb, 0x80, 0x4f, 0x80, 0x08,
0x4d, 0x1b, 0x05, 0xae,
0xca, 0xc4, 0x8a, 0x63, 0xc1, 0x03, 0x84, 0x0e, 0x1d, 0xa2, 0xcd, 0x99,
0x32, 0x65, 0x42, 0x95,
0x42, 0x05, 0x01, 0xa0, 0x1c, 0x04, 0x0c, 0x18, 0xb4, 0x78, 0xa8, 0x32,
0x67, 0xc8, 0x94, 0x08,
0x55, 0x0a, 0x54, 0x0f, 0x80, 0x6a, 0x11, 0x11, 0x08, 0x42, 0x85, 0x50,
0xd3, 0xa2, 0x44, 0xa9,
0x52, 0xa1, 0x22, 0x10, 0x54, 0xe1, 0x48, 0x00, 0x1d, 0xc1, 0xa1, 0x5a,
0xa9, 0xaa, 0x70, 0x0c,
0x0f, 0x55, 0xa0, 0x82, 0x32, 0x98, 0xc0, 0x21, 0x42, 0x55, 0x0c, 0xba,
0x74, 0xc1, 0x82, 0xa5,
0x2b, 0x3f, 0x03, 0xc0, 0x15, 0x45, 0x8a, 0x41, 0x49, 0x8b, 0xa6, 0x6b,
0xd7, 0xa1, 0x10, 0xc0,
0xae, 0xe9, 0xd1, 0x86, 0x08, 0x15, 0x2a, 0x3c, 0xad, 0x50, 0x5d, 0xb9,
0x88, 0x40, 0x07, 0x10,
0xe5, 0x05, 0xa0, 0x54, 0xa9, 0xf2, 0xfc, 0xc9, 0x73, 0xe1, 0x82, 0x96,
0x6f, 0x8a, 0xfa, 0xc2,
0x29, 0x70, 0x5c, 0xd1, 0x92, 0x45, 0xd5, 0xab, 0xbf, 0xe8, 0x88, 0x8d,
0x44, 0x0e, 0x0f, 0x5d,
0xbe, 0x28, 0x48, 0x94, 0x28, 0x5b, 0x82, 0xc4, 0x48, 0x92, 0x98, 0x09,
0x3e, 0xc2, 0x43, 0x97,
0x2c, 0x49, 0xb2, 0xe3, 0x51, 0xb6, 0x7c, 0xa9, 0x12, 0xe5, 0xcb, 0xf3,
0x21, 0x03, 0x56, 0x0f,
0xc8, 0x94, 0x59, 0x1a, 0x12, 0xfd, 0x7b, 0x0c, 0x87, 0xf0, 0xf4, 0x01,
0x43, 0x86, 0x0c, 0x59,
0xb2, 0x13, 0x20, 0x1e, 0xb2, 0x21, 0x3c, 0xd2, 0x43, 0x46, 0x78, 0xc9,
0x92, 0x19, 0x5a, 0x81,
0x10, 0x19, 0xbe, 0x64, 0x64, 0x84, 0x0c, 0xc9, 0x92, 0x65, 0x87, 0xaf,
0x7c, 0x45, 0x8f, 0xa0,
0xc1, 0xa9, 0x45, 0x0b, 0x96, 0x18, 0x59, 0xb7, 0x64, 0xc9, 0xba, 0x05,
0x06, 0xbc, 0xbe, 0x40,
0xb5, 0x02, 0x8d, 0x4e, 0x43, 0x83, 0x37, 0x6b, 0xee, 0xbc, 0xb9, 0x03,
0x02, 0xc0, 0x01, 0x39,
0xb0, 0xf0, 0x50, 0xad, 0xd5, 0x39, 0xed, 0x00, 0x38, 0x8d, 0x80, 0x70,
0x4e, 0x5d, 0x83, 0x6e,
0xd0, 0x0a, 0x14, 0x28, 0xd5, 0xed, 0xda, 0x76, 0x80, 0x6a, 0x0c, 0x00,
0x84, 0xa0, 0x95, 0x2a,
0x8c, 0x30, 0xd6, 0xfa, 0xe8, 0x69, 0x45, 0x08, 0x14, 0xa0, 0x42, 0xa5,
0x10, 0x69, 0x87, 0x06,
0xeb, 0xc8, 0x84, 0x0a, 0x54, 0xa9, 0x52, 0x70, 0xe0, 0x54, 0x2b, 0x55,
0x97, 0x1f, 0x09, 0x65,
0x55, 0x65, 0x6a, 0xa8, 0x57, 0x18, 0x01, 0xea, 0xd4, 0x01, 0xac, 0x08,
0xdb, 0x0c, 0xad, 0xda,
0x56, 0xa9, 0x52, 0x75, 0x7a, 0x24, 0x00, 0xc0, 0x68, 0x40, 0x58, 0x08,
0x56, 0x2d, 0xda, 0x0a,
0x0f, 0x90, 0xe8, 0x01, 0x82, 0x43, 0x0a, 0x12, 0x64, 0x65, 0x3a, 0x64,
0xc7, 0x80, 0x55, 0x78,
0xa2, 0x0c, 0x0f, 0x84, 0xe0, 0xc3, 0x53, 0x35, 0x55, 0xaa, 0x16, 0xe2,
0x0b, 0x65, 0x7a, 0xa0,
0x06, 0x0a, 0x5e, 0xb5, 0x42, 0x85, 0x5c, 0x5d, 0xc7, 0x0f, 0x7a, 0xa4,
0xba, 0xd1, 0x83, 0xaf,
0x41, 0x70, 0xd0, 0x5d, 0xe5, 0x97, 0x4e, 0x94, 0xe8, 0xc2, 0x13, 0x88,
0xba, 0xce, 0x40, 0xe0,
0x2a, 0xbf, 0x74, 0xa2, 0xd6, 0x0a, 0xb3, 0x32, 0x40, 0x2a, 0x8c, 0x00,
0x05, 0xb4, 0x5c, 0xc5,
0x97, 0x6e, 0x8d, 0xb0, 0x35, 0xa2, 0x07, 0xa9, 0x5a, 0x9d, 0x42, 0x55,
0xba, 0x5c, 0xd9, 0xb5,
0x60, 0x37, 0x80, 0xed, 0x59, 0xb5, 0xcb, 0x5d, 0xa9, 0x30, 0x01, 0x62,
0xa1, 0x41, 0x83, 0xc7,
0x8e, 0x5d, 0x18, 0x58, 0x0d, 0x02, 0xbe, 0xec, 0x76, 0x42, 0xa9, 0xc0,
0xb8, 0xb1, 0x6b, 0x2d,
0x22, 0xdc, 0x5a, 0x85, 0xaa, 0x4c, 0xac, 0xd8, 0xf0, 0x64, 0xcb, 0x36,
0x1c, 0xc2, 0x83, 0x69,
0x26, 0x70, 0x55, 0xab, 0x4c, 0x58, 0x48, 0xc4, 0x61, 0x78, 0x5b, 0xb3,
0xc7, 0x51, 0x6d, 0x7c,
0xc4, 0x46, 0x7c, 0x24, 0x84, 0x35, 0x66, 0xf1, 0xc1, 0x8c, 0x1d, 0x40,
0xb5, 0xc2, 0x84, 0x31,
0x8b, 0x0f, 0x66, 0xcc, 0xc2, 0x9b, 0x1b, 0x3f, 0x80, 0x62, 0xc3, 0x33,
0x0c, 0x02, 0x28, 0xbe,
0xf8, 0x71, 0x14, 0x1a, 0x1f, 0x21, 0x11, 0x10, 0x81, 0xcc, 0x35, 0x00,
0xb8, 0x0d, 0x40, 0xfc,
0x26, 0x45, 0x07, 0xb2, 0xe5, 0x38, 0x01, 0x62, 0xe3, 0x24, 0x28, 0xf9,
0x71, 0xe5, 0x0b, 0x54,
0x5d, 0x01, 0x58, 0xa3, 0x1b, 0x00, 0x54, 0x03, 0x88, 0x22, 0x35, 0x68,
0x40, 0xc7, 0x61, 0x3c,
0xf9, 0x4e, 0x12, 0x30, 0xee, 0x1c, 0x99, 0x71, 0x6b, 0x8f, 0xfe, 0xb0,
0x36, 0x80, 0xc0, 0x01,
0x70, 0x67, 0x66, 0x9d, 0x2b, 0x30, 0x60, 0x73, 0xd1, 0x1e, 0x7c, 0x81,
0x33, 0x07, 0x1b, 0x0e,
0x1c, 0x00, 0x30, 0x50, 0xaa, 0x2c, 0x1c, 0xfa, 0x43, 0x6c, 0x6c, 0x70,
0xe0, 0x06, 0x36, 0x00,
0xb8, 0x71, 0x54, 0xb7, 0x07, 0xca, 0xc4, 0x89, 0x15, 0xee, 0x43, 0x46,
0x00, 0x49, 0x96, 0x2d,
0x51, 0xb6, 0x7c, 0xf9, 0x12, 0x03, 0x41, 0x7e, 0xf8, 0xca, 0x92, 0x1a,
0x9a, 0xf2, 0xfd, 0x07,
0x80, 0x8f, 0x04, 0x91, 0x25, 0x2f, 0x0c, 0xe0, 0xd3, 0x0f, 0x00, 0xf8,
0x71, 0x90, 0xd1, 0x52,
0xe4, 0xc1, 0x0e, 0x0d, 0xfd, 0xe8, 0xe5, 0x87, 0xa7, 0x1c, 0x3f, 0x85,
0xe8, 0x83, 0x36, 0x6c,
0xf9, 0xe4, 0xd5, 0x87, 0x83, 0x4f, 0x39, 0xf1, 0x11, 0x40, 0x34, 0x74,
0x11, 0x55, 0x9d, 0x11,
0x30, 0xf3, 0xd3, 0x8f, 0x1f, 0x18, 0x11, 0x09, 0x43, 0x97, 0x6e, 0xa8,
0xf0, 0xcb, 0x51, 0x3e,
0x1c, 0x37, 0x70, 0x3a, 0x89, 0x86, 0x23, 0xaa, 0x50, 0x3d, 0xfa, 0xf3,
0xaa, 0x55, 0x2a, 0x1c,
0x38, 0x7e, 0x06, 0x41, 0x05, 0x09, 0x59, 0x5e, 0xe1, 0xc1, 0x83, 0xef,
0xd1, 0x8b, 0x2f, 0x99,
0x6e, 0x3a, 0x48, 0x85, 0x2a, 0xd5, 0xf2, 0xfc, 0xf9, 0x93, 0xef, 0xd1,
0xbf, 0x47, 0x79, 0x79,
0xd8, 0x07, 0xaa, 0x54, 0xaa, 0x53, 0x27, 0x31, 0x3c, 0xfc, 0xcb, 0x8b,
0x8a, 0xf1, 0xf0, 0xe5,
0x1b, 0xa2, 0x6e, 0xa5, 0xe1, 0xa1, 0x40, 0x5e, 0x19, 0xf8, 0x97, 0x2a,
0x71, 0x3e, 0x7c, 0xfb,
0xd6, 0xac, 0x44, 0x89, 0x6a, 0xa5, 0xaa, 0xd5, 0x6d, 0xd4, 0x7e, 0xf8,
0xf0, 0x24, 0x5b, 0xa6,
0x4a, 0x9c, 0x4a, 0x44, 0xaa, 0x53, 0xad, 0x30, 0x7c, 0x7c, 0x5d, 0x03,
0x46, 0x85, 0xea, 0xd5,
0x2b, 0x55, 0xa4, 0x30, 0x82, 0xce, 0x43, 0xa2, 0x44, 0x99, 0xf8, 0x55,
0xaa, 0x57, 0xa8, 0x4e,
0xa1, 0x12, 0x85, 0xe4, 0x62, 0x41, 0xa5, 0x4a, 0x94, 0x3a, 0x51, 0xea,
0xd6, 0x09, 0x1b, 0x3d,
0xe8, 0x02, 0x50, 0xad, 0x00, 0x95, 0xab, 0x14, 0x40, 0x1f, 0x9e, 0xba,
0xe1, 0x03, 0x05, 0x0d,
0xba, 0x20, 0x54, 0xa9, 0xa2, 0x95, 0x13, 0xf1, 0xa9, 0x5f, 0x37, 0x55,
0xd4, 0x80, 0xc0, 0x15,
0x44, 0x78, 0xc0, 0x52, 0x9f, 0x1e, 0x3c, 0x51, 0x6a, 0x84, 0x49, 0x11,
0xa9, 0x56, 0xa4, 0x0a,
0x15, 0xc4, 0x87, 0xae, 0xf0, 0xe0, 0xa9, 0x13, 0xbd, 0x7e, 0x9a, 0xb4,
0x91, 0xea, 0xd6, 0x9a,
0x0f, 0xe1, 0xa9, 0x12, 0x24, 0x4f, 0xfe, 0x08, 0xc3, 0x83, 0x26, 0x3a,
0xdd, 0xfa, 0xf2, 0x51,
0x15, 0xa8, 0x7a, 0x40, 0x19, 0x0e, 0xf0, 0x69, 0xc2, 0x44, 0x0a, 0x51,
0x9f, 0xf2, 0xf4, 0x3c,
0x89, 0x78, 0x48, 0x87, 0xf0, 0xd0, 0xa9, 0x91, 0x1e, 0x52, 0x45, 0xe1,
0x05, 0x32, 0x2d, 0xc3,
0x0b, 0x3e, 0x3d, 0xa0, 0x00, 0xe2, 0x51, 0x15, 0x7a, 0x84, 0xf1, 0xa0,
0x9f, 0x3e, 0x4c, 0x9d,
0x14, 0xf5, 0x81, 0x06, 0x15, 0x5e, 0xe1, 0x90, 0x8e, 0xee, 0x61, 0xea,
0xa6, 0x07, 0x0e, 0x78,
0x3c, 0x29, 0x08, 0x0f, 0xfe, 0xf1, 0xe0, 0x3e, 0x1e, 0x91, 0xa6, 0xb3,
0xa2, 0x78, 0x86, 0x0d,
0x15, 0x2a, 0x52, 0x84, 0x00, 0x40, 0x65, 0x80, 0x02, 0x45, 0xa8, 0xc3,
0x83, 0x3e, 0x7d, 0xf8,
0x54, 0xfb, 0x82, 0x37, 0x02, 0x54, 0x05, 0xa0, 0x48, 0xd1, 0x6a, 0xe8,
0x77, 0x1f, 0x0e, 0x1a,
0x35, 0x6a, 0x45, 0x8d, 0x4a, 0x17, 0x8a, 0xf0, 0x8a, 0x47, 0xfa, 0xf0,
0xd6, 0x40, 0xa3, 0x76,
0x5d, 0x3a, 0x40, 0xa4, 0x83, 0x22, 0x8a, 0x14, 0xa9, 0x06, 0x00, 0x5d,
0x6a, 0xd4, 0xa8, 0x16,
0x02, 0x34, 0x70, 0xc2, 0x84, 0x0a, 0xe4, 0xcb, 0x2d, 0x00, 0xf8, 0x06,
0x21, 0x5f, 0xae, 0x5c,
0x81, 0x85, 0xa7, 0x30, 0x76, 0x6c, 0xd9, 0x32, 0x0b, 0x40, 0xe6, 0xcc,
0x98, 0xb2, 0x63, 0x06,
0x54, 0x59, 0x78, 0xf0, 0x8b, 0xa7, 0x04, 0xe2, 0xca, 0x0f, 0x18, 0x50,
0xa5, 0x42, 0x85, 0xc7,
0x67, 0x02, 0x58, 0x0b, 0xc0, 0xf8, 0x0a, 0x8f, 0xf4, 0xe0, 0x1b, 0x81,
0x6c, 0xe3, 0x8b, 0x2b,
0x37, 0x60, 0xe1, 0xc9, 0x94, 0xa9, 0xdd, 0x78, 0xb2, 0x9e, 0x1f, 0x4c,
0xc3, 0xd3, 0x9c, 0x30,
0x8e, 0x05, 0x18, 0x50, 0xdc, 0xe3, 0x4b, 0x68, 0x7d, 0xb0, 0x6e, 0xc0,
0x3c, 0xa8, 0x4f, 0x76,
0x4c, 0x99, 0x01, 0x2f, 0x0f, 0xb1, 0xbc, 0x1b, 0xa0, 0xbe, 0x86, 0x81,
0x5f, 0x7f, 0x00, 0x8f,
0x0f, 0xe1, 0xbc, 0x38, 0x16, 0x47, 0x00, 0x8d, 0x01, 0xb8, 0xf0, 0x76,
0xe5, 0x9a, 0x1e, 0x45,
0x68, 0x01, 0x00, 0x17, 0x9a, 0x36, 0x86, 0xe9, 0x21, 0x4c, 0xac, 0x40,
0x5b, 0xb6, 0xd8, 0x69,
0xe3, 0xce, 0x80, 0xbb, 0xdd, 0x86, 0x60, 0xa8, 0x4c, 0xb8, 0x50, 0xb3,
0x62, 0x59, 0xb1, 0x62,
0xca, 0x1a, 0x2e, 0x5d, 0xbb, 0x03, 0x01, 0xdc, 0xa2, 0x35, 0xee, 0xf9,
0x61, 0xd4, 0x96, 0x03,
0x77, 0x4e, 0xed, 0x72, 0x63, 0x46, 0x1d, 0x98, 0xb2, 0xf1, 0x50, 0x50,
0x0f, 0x62, 0x69, 0xc1,
0x66, 0xa7, 0x4f, 0x9b, 0x03, 0x06, 0xcc, 0xa9, 0x59, 0xb3, 0x6a, 0xad,
0x3f, 0xc4, 0x0a, 0xa5,
0xe1, 0x4b, 0xa2, 0x7c, 0xf9, 0xea, 0x03, 0x50, 0x9d, 0x6a, 0xa5, 0x2a,
0x15, 0x86, 0x06, 0x0c,
0x1f, 0xbe, 0x23, 0x40, 0xbd, 0x7e, 0xfa, 0x14, 0xdd, 0xd1, 0x86, 0x08,
0x5a, 0x95, 0x52, 0xd9,
0x3a, 0x7c, 0xf8, 0x92, 0x27, 0x1f, 0xa6, 0x7b, 0xf7, 0x2e, 0x1d, 0xba,
0x77, 0xe8, 0x5a, 0x83,
0x4a, 0xa5, 0x34, 0x7c, 0xf8, 0xf4, 0xe7, 0x33, 0x1c, 0xc2, 0xc2, 0x69,
0x40, 0x38, 0x74, 0x09,
0x41, 0x75, 0x78, 0xf8, 0xf2, 0x2a, 0x5f, 0x22, 0xbd, 0x26, 0x70, 0x6f,
0xd3, 0xa9, 0x6d, 0x8b,
0x14, 0x75, 0xd3, 0x71, 0xe1, 0xcb, 0xb7, 0xbc, 0x00, 0x70, 0xe7, 0xda,
0xa1, 0x55, 0x76, 0xbc,
0x39, 0x31, 0x82, 0xa8, 0x23, 0x01, 0x7d, 0x4b, 0x92, 0xa7, 0xcf, 0x9d,
0x6d, 0x5e, 0xbc, 0x18,
0x42, 0xd4, 0x8d, 0x48, 0x0f, 0x1c, 0x17, 0x15, 0x21, 0x41, 0xaa, 0x56,
0xd7, 0x30, 0x61, 0x42,
0xd1, 0xac, 0x5b, 0xab, 0x6e, 0x1d, 0xb0, 0x06, 0xc2, 0x97, 0x24, 0xa9,
0x30, 0xf5, 0xc3, 0xd7,
0xaf, 0x1b, 0x26, 0x64, 0xd7, 0x4e, 0xe9, 0xa8, 0x90, 0x31, 0x08, 0xbe,
0xa4, 0x86, 0x06, 0x4d,
0x97, 0x01, 0x60, 0x93, 0x89, 0x6d, 0x3d, 0x30, 0x74, 0xc8, 0x20, 0x21,
0xcb, 0xa3, 0x7c, 0xff,
0x34, 0x6d, 0xea, 0xd4, 0xe2, 0xc5, 0x8b, 0x16, 0x19, 0x7a, 0x7c, 0xb8,
0x80, 0x01, 0x4b, 0xab,
0x7e, 0xf9, 0xb0, 0x7d, 0x06, 0x40, 0x44, 0x48, 0x91, 0x11, 0x17, 0x30,
0x64, 0x00, 0xd0, 0x07,
0x9f, 0x66, 0x7a, 0x05, 0x01, 0x94, 0xf0, 0xd4, 0xa1, 0x63, 0x3b, 0xd4,
0xab, 0x04, 0x19, 0x00,
0x2a, 0x55, 0x2a, 0x01, 0x22, 0x27, 0x01, 0x74, 0xc0, 0x50, 0xa5, 0xe0,
0x36, 0x54, 0xaa, 0x7e,
0xca, 0xa0, 0x38, 0x8f, 0xf0, 0x54, 0xa1, 0x4a, 0xb4, 0x50, 0x85, 0x4a,
0xd5, 0x09, 0x14, 0xa8,
0x54, 0xa1, 0x4e, 0xfd, 0xee, 0xe9, 0xc3, 0xd7, 0xa7, 0x3b, 0x20, 0x34,
0xe8, 0x42, 0x05, 0x0a,
0x90, 0x22, 0x45, 0xe1, 0xe1, 0x10, 0xbe, 0xc6, 0x69, 0xd0, 0x07, 0x5b,
0x97, 0x2e, 0x1e, 0x1a,
0xc2, 0x8b, 0x26, 0x44, 0xfd, 0x1a, 0xa1, 0xc2, 0xd3, 0xaa, 0x3d, 0x3c,
0x74, 0x85, 0x27, 0xa0,
0xe9, 0x80, 0x08, 0x1f, 0x2a, 0x54, 0xad, 0x1a, 0x35, 0x85, 0x27, 0xa2,
0xf8, 0xa0, 0x0d, 0x85,
0x26, 0x7c, 0xf8, 0xf4, 0x60, 0xc3, 0xbb, 0x89, 0x02, 0x8c, 0x07, 0x4d,
0xae, 0x29, 0x95, 0x45,
0x3e, 0x06, 0x14, 0x6a, 0xd0, 0x80, 0xc2, 0xc1, 0x35, 0x6d, 0x9d, 0xb0,
0xa9, 0xea, 0xd6, 0xa8,
0x3b, 0x37, 0x34, 0x82, 0x06, 0x10, 0x8e, 0x0e, 0x21, 0xd2, 0x4c, 0x03,
0xd8, 0x10, 0x61, 0xb5,
0x25, 0x40, 0xda, 0xce, 0xc3, 0xc1, 0x29, 0x5d, 0x98, 0x1a, 0x35, 0xf6,
0x43, 0x5f, 0x80, 0x42,
0x45, 0xcb, 0xa9, 0xc3, 0x7f, 0x70, 0xfa, 0x03, 0xf4, 0xa8, 0x96, 0x25,
0x2a, 0x14, 0xb4, 0x1c,
0x84, 0x45, 0x3c, 0x38, 0x9c, 0x0f, 0x7d, 0xbd, 0x89, 0x4a, 0x57, 0x38,
0xa6, 0x85, 0xbb, 0xc0,
0x58, 0x09, 0x8d, 0xa8, 0x02, 0x00, 0x96, 0x83, 0x70, 0x0c, 0x0f, 0xd7,
0x14, 0xa1, 0xba, 0x73,
0xe8, 0xc0, 0x15, 0x2d, 0x57, 0xe1, 0xe0, 0x28, 0xbc, 0xd1, 0xe9, 0x0b,
0x06, 0x47, 0xae, 0x02,
0x20, 0x9c, 0x9d, 0xba, 0x86, 0x07, 0x4e, 0x5f, 0x18, 0x44, 0x60, 0xb8,
0x38, 0xa2, 0xaa, 0x4e,
0x1f, 0xb8, 0x38, 0x4c, 0xcb, 0x70, 0x2e, 0x07, 0x75, 0xf1, 0x81, 0x8a,
0x2b, 0x3b, 0x7d, 0xda,
0x80, 0x51, 0x63, 0xc6, 0xd6, 0x99, 0x55, 0x6b, 0x5c, 0xd5, 0x0a, 0x13,
0xaa, 0x1a, 0x1c, 0x3b,
0xbd, 0x60, 0xf5, 0xea, 0x75, 0xcb, 0x96, 0xae, 0x56, 0x85, 0xe1, 0x60,
0x54, 0x80, 0x3a, 0x75,
0xec, 0xb4, 0x26, 0x23, 0x3d, 0x7b, 0x4c, 0xdd, 0x81, 0x13, 0x26, 0xcc,
0xb4, 0xa8, 0xf0, 0xd4,
0xae, 0x4c, 0x59, 0x02, 0xd0, 0xb5, 0x06, 0x0f, 0x6a, 0x5a, 0x08, 0x30,
0x21, 0x4e, 0x5c, 0x7e,
0x24, 0x46, 0x0d, 0x38, 0x65, 0xca, 0xce, 0x99, 0x5a, 0x81, 0xa2, 0x44,
0x89, 0x6b, 0x0c, 0x7d,
0xca, 0x05, 0x28, 0xd7, 0x06, 0x8b, 0x82, 0x3d, 0x6a, 0x5a, 0x45, 0x87,
0xa7, 0x38, 0xad, 0xfa,
0x54, 0xab, 0x52, 0x8e, 0x4c, 0x97, 0xeb, 0xfa, 0xd0, 0x27, 0x20, 0xbc,
0xb8, 0xc3, 0x53, 0x28,
0x4a, 0xb9, 0x72, 0x5d, 0x14, 0x99, 0xd9, 0xd5, 0x2a, 0x4c, 0x94, 0x08,
0x75, 0xaa, 0x79, 0xc1,
0x53, 0x1a, 0x1f, 0x0a, 0x74, 0x51, 0xa5, 0xc6, 0x96, 0x9f, 0xc0, 0x04,
0x08, 0x0d, 0x50, 0xf4,
0xc2, 0x8b, 0x41, 0x39, 0xb0, 0xe5, 0x2d, 0x50, 0x98, 0x80, 0xdc, 0x08,
0x0f, 0x70, 0xaa, 0x84,
0x29, 0x60, 0x0d, 0x15, 0x1a, 0x53, 0xd6, 0x02, 0x84, 0x0a, 0x07, 0xad,
0x4a, 0x55, 0x78, 0xe6,
0x43, 0x78, 0x68, 0xa3, 0xca, 0x40, 0x94, 0x68, 0x81, 0x1c, 0xc3, 0x93,
0x5f, 0x7d, 0x30, 0x60,
0x0d, 0x34, 0x3c, 0x55, 0x89, 0x12, 0x4a, 0x11, 0x94, 0x6a, 0x78, 0xfc,
0x14, 0x70, 0x60, 0xc5,
0x5b, 0xa9, 0x5e, 0xa8, 0xb6, 0xc2, 0x43, 0x34, 0x44, 0x56, 0xa0, 0xe1,
0xe9, 0x53, 0xc4, 0x80,
0x95, 0xea, 0xf0, 0xd0, 0x0a, 0x2b, 0x3e, 0x44, 0x81, 0xa3, 0x15, 0x9e,
0x00, 0x68, 0xf1, 0x52,
0xa8, 0x14, 0x98, 0x3e, 0x5a, 0xe1, 0x49, 0x03, 0x86, 0x1f, 0x1d, 0x3a,
0x54, 0x88, 0x50, 0xa5,
0x5a, 0xe1, 0x4b, 0xb4, 0x86, 0x62, 0xd5, 0x2a, 0xe0, 0xc0, 0xf0, 0x11,
0x1e, 0x2a, 0x54, 0x05,
0x84, 0xea, 0x5f, 0x68, 0x0d, 0x50, 0x2a, 0x48, 0xe8, 0x70, 0x91, 0x00,
0x3a, 0x78, 0xa0, 0x40,
0x01, 0xe0, 0x17, 0x14, 0xf4, 0x81, 0x9a, 0xd8, 0x00, 0x91, 0x01, 0x34,
0x02, 0x00, 0x86, 0x8e,
0x1b, 0xd0, 0xcd, 0x8b, 0x47, 0x2e, 0x88, 0xc0, 0x21, 0x22, 0x08, 0x54,
0xc8, 0xd0, 0x01, 0x8b,
0x22, 0x3d, 0xd8, 0x3a, 0x74, 0x91, 0x08, 0x40, 0x1c, 0x01, 0x10, 0x09,
0x05, 0x08, 0x4e, 0x23,
0x64, 0x2a, 0xe1, 0x14, 0x19, 0x84, 0x49, 0x11, 0xc6, 0x55, 0x01, 0xe2,
0x04, 0x2a, 0x51, 0x12,
0x9a, 0x84, 0x89, 0x04, 0x60, 0x44, 0x90, 0x22, 0x88, 0x0f, 0xa3, 0x10,
0x21, 0xf1, 0x11, 0x56,
0x81, 0x4d, 0x90, 0x1c, 0x41, 0x2c, 0xe1, 0x1f, 0x95, 0x44, 0xc3, 0x13,
0x4b, 0xf8, 0x13, 0x21,
0x49, 0x2e, 0x3e, 0x52, 0x23, 0x1f, 0xd2, 0x23, 0x2a, 0x02, 0x0a, 0x37,
0x3e, 0xa2, 0xa4, 0xc3,
0x2b, 0x2c, 0xf2, 0x15, 0x1f, 0x3e, 0x9c, 0xe9, 0x89, 0xb9, 0x7c, 0x49,
0xe7, 0x03, 0x56, 0x9c,
0xb5, 0x81, 0x23, 0x3d, 0x3b, 0x9a, 0x20, 0x51, 0xa2, 0xb8, 0x53, 0x32,
0xbf, 0x22, 0x3a, 0x3e,
0x08, 0x92, 0x8c, 0x45, 0x57, 0x01, 0xe8, 0xc0, 0x75, 0x00, 0x38, 0x0d,
0x0c, 0x77, 0xee, 0x3e,
0x42, 0x9f, 0x83, 0x08, 0xa0, 0x10, 0x2e, 0xf0, 0xe8, 0xb9, 0xd3, 0xf7,
0x09, 0x61, 0x01, 0x00,
0xb6, 0x6b, 0x87, 0x4e, 0x9d, 0x3a, 0x08, 0x83, 0xf0, 0x70, 0x47, 0x0f,
0x9e, 0x26, 0x42, 0xc4,
0x75, 0x87, 0x93, 0x53, 0x77, 0xf2, 0xe0, 0xc5, 0x17, 0x69, 0xe2, 0xe1,
0xe9, 0xce, 0x41, 0x20,
0x84, 0x87, 0xbe, 0xf4, 0xe4, 0x5d, 0x15, 0xa4, 0x35, 0xba, 0x73, 0x54,
0x9a, 0xe1, 0x05, 0x4f,
0x35, 0x14, 0x52, 0x04, 0x70, 0x6b, 0xac, 0xa5, 0xf8, 0x94, 0xe7, 0x94,
0x36, 0x10, 0x2d, 0x38,
0x70, 0xe0, 0x92, 0xe5, 0x28, 0x9c, 0xc2, 0x43, 0xa9, 0x4b, 0x27, 0x50,
0x14, 0x87, 0x27, 0x81,
0x70, 0x76, 0x27, 0x51, 0x25, 0x14, 0x27, 0x90, 0x15, 0x63, 0xce, 0x00,
0xa2, 0xe1, 0xed, 0x10,
0x89, 0x12, 0x2d, 0x8e, 0x35, 0x87, 0x07, 0xe9, 0x6f, 0xe8, 0xc1, 0x70,
0x70, 0xa2, 0x45, 0x89,
0xe6, 0xf0, 0xed, 0x01, 0x77, 0xee, 0x9d, 0xc4, 0x26, 0x60, 0xc7, 0x90,
0x05, 0xc7, 0x07, 0x51,
0x7c, 0x23, 0x10, 0x17, 0x8e, 0xe3, 0x83, 0x88, 0x62, 0xca, 0x8e, 0x15,
0x63, 0xc1, 0x82, 0xf5,
0x08, 0xdc, 0xb9, 0x76, 0x12, 0x1f, 0x50, 0x24, 0x2b, 0xce, 0xc0, 0xf0,
0xc0, 0x18, 0x9e, 0x4e,
0xd3, 0x21, 0x00, 0xa1, 0x68, 0xa6, 0x4c, 0x39, 0x3e, 0x70, 0x2b, 0x0d,
0xcf, 0x5e, 0x08, 0x00,
0x57, 0x4e, 0x5c, 0x46, 0x85, 0x3a, 0xdd, 0x8c, 0x98, 0xea, 0x63, 0x00,
0x0b, 0x94, 0x42, 0xa5,
0xca, 0xd8, 0xc1, 0x52, 0x25, 0x4a, 0xd4, 0x47, 0x50, 0xa4, 0x47, 0x8f,
0xb6, 0x6e, 0x45, 0x4a,
0xc3, 0x43, 0x1f, 0xab, 0xf0, 0x02, 0x07, 0x8f, 0x1d, 0x3b, 0x7a, 0xfa,
0x02, 0x40, 0xab, 0x5e,
0x78, 0xe1, 0x65, 0xd5, 0x1e, 0x3d, 0x7b, 0x11, 0x00, 0x2f, 0xbc, 0x99,
0x2a, 0x14, 0x28, 0x90,
0x1d, 0x43, 0xd6, 0xe1, 0xc1, 0x94, 0x5d, 0x36, 0x28, 0x55, 0xab, 0x95,
0x2b, 0x58, 0x61, 0xc2,
0x14, 0xaa, 0x06, 0x40, 0x8f, 0x29, 0x2b, 0x26, 0xaa, 0x54, 0x63, 0x0d,
0x0f, 0xde, 0xf9, 0xa9,
0x50, 0x11, 0x68, 0x7a, 0x14, 0xa9, 0xd0, 0x02, 0xa5, 0x5a, 0x5d, 0xf8,
0xa8, 0x56, 0xa5, 0x15,
0x1e, 0x6c, 0x26, 0x8c, 0x61, 0x85, 0xa7, 0x5e, 0x8e, 0xe1, 0x0d, 0x95,
0x1d, 0x6b, 0x26, 0x5c,
0x20, 0x6b, 0x89, 0x0f, 0x6d, 0x40, 0xe3, 0x4b, 0x17, 0x43, 0x7e, 0xe1,
0x01, 0x98, 0x31, 0x2b,
0xa0, 0xe1, 0xc1, 0x4f, 0x65, 0x7c, 0x80, 0x66, 0xa5, 0x11, 0x1c, 0x4b,
0x26, 0x40, 0x80, 0x04,
0x03, 0x50, 0xbd, 0x5c, 0x55, 0x97, 0x07, 0x6f, 0x56, 0x1c, 0xc1, 0x31,
0x89, 0x8f, 0x5b, 0x60,
0xcd, 0x2f, 0xbc, 0x59, 0xb3, 0x52, 0x0a, 0x90, 0x31, 0xe3, 0xf0, 0x00,
0xcc, 0x92, 0x1d, 0x5b,
0xb6, 0x4a, 0x45, 0x09, 0x60, 0xad, 0x4b, 0x9d, 0xea, 0xf0, 0x52, 0xac,
0x2b, 0x3c, 0x98, 0x29,
0x55, 0x2e, 0x9c, 0x35, 0x20, 0x75, 0x0a, 0xc2, 0x1b, 0x94, 0x46, 0xaa,
0x6c, 0xd7, 0x43, 0x98,
0x56, 0x85, 0x0a, 0x55, 0xe9, 0x62, 0xc5, 0x59, 0xb1, 0x12, 0x25, 0xb0,
0xe9, 0xf1, 0x15, 0x26,
0x4e, 0x18, 0x09, 0xc2, 0x44, 0x02, 0x18, 0x0b, 0x11, 0x9c, 0x24, 0x43,
0x2a, 0xbc, 0x95, 0x10,
0x91, 0xa2, 0x25, 0x00, 0xa0, 0x50, 0x09, 0xc0, 0xf8, 0x08, 0xc1, 0x00,
0x64, 0xa2, 0x05, 0x49,
0x00, 0x0c, 0x03, 0x10, 0x18, 0x94, 0xa1, 0x84, 0x6c, 0x78, 0xa2, 0x84,
0x4d, 0x1b, 0x15, 0xe0,
0x50, 0x0c, 0x44, 0x90, 0xa0, 0x51, 0x23, 0xa4, 0x4a, 0x91, 0x21, 0x6a,
0x54, 0xa0, 0x40, 0x02,
0x04, 0x1d, 0x06, 0x00, 0x01, 0xa2, 0x86, 0x44, 0x89, 0x11, 0x02, 0xd4,
0xb0, 0xc3, 0x23, 0x14,
0x00, 0x22, 0x44, 0x8d, 0x08, 0x12, 0x05, 0x06, 0x08, 0x10, 0x30, 0x70,
0xe8, 0x4c, 0x17, 0x80,
0xd0, 0xd0, 0xad, 0x31, 0x1c, 0x35, 0x30, 0x80, 0x44, 0xcd, 0x91, 0x43,
0x87, 0xb4, 0x20, 0x50,
0x82, 0x04, 0x1b, 0x22, 0x24, 0x8a, 0x94, 0xa8, 0x41, 0x84, 0x07, 0x0f,
0x6a, 0x00, 0x84, 0x45,
0x00, 0x84, 0x43, 0x60, 0x06, 0x40, 0x58, 0x40, 0x0b, 0x0a, 0x4a, 0x9a,
0x10, 0x21, 0x82, 0xa6,
0x49, 0x23, 0x42, 0xad, 0xa1, 0x01, 0x55, 0xa3, 0x6e, 0x46, 0x61, 0x01,
0x28, 0x00, 0x80, 0x01,
0x02, 0x0d, 0x00, 0x10, 0x82, 0x90, 0x00, 0x10, 0x80, 0x66, 0x02, 0x4c,
0x8d, 0x2a, 0x55, 0x6a,
0x02, 0x40, 0x55, 0xf8, 0xc7, 0x80, 0x18, 0x31, 0x6a, 0x02, 0x21, 0xfe,
0x23, 0x28, 0xcd, 0xb3,
0xde, 0x0c, 0xed, 0x00, 0xa0, 0x48, 0x8f, 0x9e, 0x3b, 0xd7, 0x01, 0xe0,
0x68, 0x2c, 0x9c, 0x06,
0xc4, 0x5a, 0x80, 0x06, 0x0d, 0x0e, 0x1c, 0xbc, 0x08, 0x8a, 0x24, 0x47,
0x80, 0x00, 0x02, 0x05,
0x1a, 0x1e, 0xe0, 0xf4, 0xd1, 0x76, 0xe4, 0x20, 0x80, 0x01, 0x76, 0x45,
0x7c, 0x26, 0x04, 0x42,
0x44, 0x10, 0x28, 0x44, 0x54, 0x7c, 0x84, 0x55, 0x60, 0x8e, 0x25, 0x02,
0x0d, 0x10, 0x1c, 0x84,
0x0d, 0xba, 0x28, 0x00, 0xba, 0x02, 0x00, 0xc2, 0x43, 0x43, 0xb8, 0x04,
0x10, 0x32, 0x4d, 0x9f,
0x11, 0x1f, 0xa1, 0xa4, 0x2f, 0x00, 0x82, 0xf0, 0x15, 0xd2, 0xa2, 0x33,
0x10, 0x6a, 0xd4, 0xaa,
0x31, 0x00, 0xb4, 0x6a, 0xd5, 0x37, 0x04, 0x0e, 0x1d, 0x0d, 0xe5, 0x77,
0x42, 0xd4, 0x08, 0x11,
0xa1, 0x6e, 0x8a, 0x0e, 0x03, 0x88, 0x22, 0x44, 0x44, 0xa8, 0x51, 0x4f,
0x12, 0x20, 0x4d, 0x90,
0x74, 0xeb, 0x46, 0xf4, 0x29, 0x81, 0x1f, 0x46, 0xaa, 0x4c, 0x7d, 0x47,
0x80, 0x80, 0x0a, 0x00,
0x30, 0x01, 0x61, 0x22, 0x40, 0x45, 0xfc, 0x45, 0x40, 0xaa, 0x51, 0xf3,
0xff, 0x7f, 0x11, 0xc1,
0xe1, 0x1f, 0xa3, 0xfc, 0xd4, 0xa9, 0x53, 0xc8, 0x9b, 0x35, 0xaf, 0x22,
0xd0, 0xa2, 0x85, 0x2a,
0x58, 0x65, 0x42, 0x85, 0x86, 0x43, 0x40, 0xf0, 0x63, 0xc7, 0x4f, 0xa1,
0x02, 0x5d, 0xb4, 0x78,
0xeb, 0x13, 0xab, 0x56, 0x28, 0xb8, 0x08, 0x04, 0xc7, 0x4e, 0x9f, 0xba,
0xa5, 0xa0, 0xa8, 0x51,
0x9f, 0x5a, 0x65, 0xea, 0xe0, 0x85, 0x0f, 0x20, 0x0d, 0x14, 0x34, 0x51,
0xd2, 0xa8, 0x4e, 0xac,
0x40, 0xa7, 0x05, 0x18, 0x1f, 0x8c, 0x34, 0x84, 0x07, 0x07, 0x07, 0x8a,
0xf2, 0x41, 0xac, 0x3d,
0x7a, 0xb7, 0xa1, 0x8f, 0x51, 0x18, 0x50, 0x4a, 0x00, 0x0d, 0x8a, 0x54,
0x27, 0x00, 0x3b, 0x7b,
0xee, 0xe0, 0x81, 0xa6, 0x64, 0x9b, 0x12, 0x24, 0x48, 0x0e, 0x14, 0x28,
0x10, 0x24, 0x50, 0x28,
0x3c, 0x76, 0xec, 0x28, 0x5e, 0x05, 0x6d, 0x86, 0xba, 0x5d, 0x6b, 0x12,
0xa4, 0x48, 0x90, 0x20,
0xa1, 0xf4, 0xa8, 0x32, 0x64, 0xc8, 0x31, 0x00, 0x1d, 0xea, 0x56, 0xad,
0x5a, 0x74, 0x00, 0x6a,
0x84, 0x4d, 0x9b, 0x22, 0xed, 0xc0, 0x70, 0x0d, 0x1b, 0xb5, 0xa2, 0x00,
0x88, 0x0f, 0x41, 0x1a,
0x10, 0x20, 0xd0, 0x90, 0x1b, 0x10, 0x20, 0x69, 0xd2, 0xf4, 0x17, 0xe1,
0xf5, 0xff, 0x21, 0x50,
0x1e, 0x02, 0x02, 0xa0, 0x0a, 0x02, 0x02, 0x00, 0x02, 0x54, 0xa8, 0xfe,
0xe2, 0x10, 0x54, 0x89,
0x0a, 0x00, 0x53, 0x26, 0x4c, 0x99, 0x42, 0xa1, 0x23, 0x00, 0x68, 0xe8,
0x51, 0x83, 0x46, 0x0f,
0x9f, 0xcf, 0x30, 0x11, 0x1e, 0x26, 0x74, 0xa8, 0x08, 0x03, 0x3d, 0x70,
0xf4, 0x44, 0xc4, 0x3f,
0x88, 0xf9, 0x2f, 0x35, 0xb1, 0x60, 0x06, 0x8c, 0x1a, 0x33, 0xf1, 0xa9,
0xc6, 0x0c,
};
void
setup()
{
delay(250) ;
GD.begin() ;
GD.ascii() ;
// setup the color map
GD.wr16 (PALETTE16A + 0, RGB (234, 215, 180));
GD.wr16 (PALETTE16A + 2, RGB (90, 105, 78));
GD.wr16 (PALETTE16A + 4, RGB (177, 214, 153));
GD.wr16 (PALETTE16A + 6, RGB (225, 233, 240));
GD.wr16 (PALETTE16A + 8, RGB (186, 209, 238));
GD.wr16 (PALETTE16A + 10, RGB (95, 69, 43));
GD.wr16 (PALETTE16A + 12, RGB (192, 134, 82));
GD.wr16 (PALETTE16A + 14, RGB (213, 141, 154));
GD.wr16 (PALETTE16A + 16, RGB (234, 234, 237));
GD.wr16 (PALETTE16A + 18, RGB (162, 201, 215));
GD.wr16 (PALETTE16A + 20, RGB (229, 234, 224));
GD.wr16 (PALETTE16A + 22, RGB (158, 139, 154));
GD.wr16 (PALETTE16A + 24, RGB (238, 246, 242));
GD.wr16 (BG_COLOR, RGB (238, 246, 242));
GD.wr16 (PALETTE16A + 26, RGB (190, 47, 49));
GD.wr16 (PALETTE16A + 28, RGB (91, 19, 27));
GD.wr16 (PALETTE16A + 30, RGB (87, 119, 158));
GD.uncompress(RAM_SPRIMG, data) ;
int i ;
for (i = 0x20; i < 0x80; i++) {
GD.setpal(4 * i + 0, TRANSPARENT);
GD.setpal(4 * i + 3, RGB(51,102,153));
}
int x, y, s ;
for (y=0, s=0; y<128; y+=16) {
for (x=0; x<96; x+=32, s++) {
GD.sprite(2*s, x+32, y+32, s, 4, 0, 0) ;
GD.sprite(2*s+1, x+48, y+32, s, 6, 0, 0) ;
}
}
GD.putstr(25, 12, "Brainwagon Demo") ;
}
void
loop()
{
for (;;) ;
}
[/sourcecode]